The group of MATLAB functions offered by the toolbox that a developer needs to know, can be divided into three groups:
- Functions to configure and execute the libraries.
- Functions to handle the configuration parameters
- Auxiliary functions.
The functions in the first two groups have the mhtb_ (metaheuristics toolbox) prefix to distinguish them from the auxiliary functions and the ones defined by the user. If you want to add your library to our toolbox, you will need to know these functions and you will need to implement the mhtb_specific function. The help MATLAB command followed by the name of any of the functions presented in this section can be used at any time to get information about them.
1. Functions to Configure and Execute the Libraries
This group is formed by four functions: mhtb_main, mhtb_specific, mhtb_launchServers and mhtb_stopServers. The first one, mhtb_main, is explained in the user’s guide. The relevant thing that a developer needs to know about this function is that it receives the configuration parameters of the library with a given format. The second one, mhtb_specific, must be implemented by you when integrating a new library. That is why it will be explained in detail.
mhtb_specific(options, origdir)
All the libraries need one function with this name and syntax to configure and execute them. It will have to deal with the configuration parameters and it will have to launch and stop the evaluation server when the user so decides. We encourage you to use the functions explained in this guide to that end.
- options: It is a cell matrix containing the configuration parameters of the algorithm, received from mhtb_main.
- origdir: It contains the path to the directory from which mhtb_main has been called. When the execution of the library ends or an error occurs, MATLAB comes back to this directory.
This function must be located in a direct subdirectory of the root of the toolbox. mhtb_main must know the location of this specific function to be able to call it. To make that possible, you must register the new library in the libraries.txt file. This file, located in the root directory of the toolbox, has one line for each library in the toolbox, joining the names of these libraries to the directories in which their specific functions are found. Table 1 shows the contents of the file for the three integrated libraries.
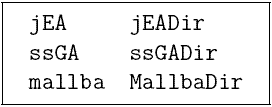
Table 1: Contents of libraries.txt.
To register a new library you must add another line containing a library ID and, separated by blanks, the directory in which the specific function is located.
Let’s imagine you want to add a library identified as MyLibrary with its specific function located in MyLibDir. Once this information is added to the file, the result will be like this:
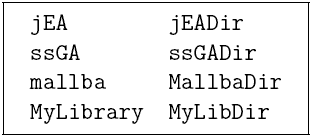
Table 1: Contents of libraries.txt after the addition of a new library. .
There are some points you must take into account when implementing mhtb_specific:
- It must accept and correctly process all the toolbox general options shown in Section 5 of the user’s guide. You can define all the particular options you wish, but they must adjust to the given format. To deal with the configuration parameters, we recommend you to use the available functions aimed to that end.
- It must launch and stop the evaluation server when necessary. To this end, you are advised to use the mhtb_launchServers and mhtb_stopServers functions.
If the library is implemented in Java, you must edit the classpath.txt file as explained in Section 3 of the user’s guide, to allow MATLAB to find the required classes.
mhtb_launchServers(localeval, typeexec, serverlocation, serverip, serverevalport, servercontrolport, options, origdir)
This function launches the evaluation server(s) when the user sets the corresponding option.
- localeval: It is a variable that can take the values 0, 1 or 2. If it is set to 0, this function will only launch one instance of the server. All the processes participating in the problem solution, no matter the machine they are running in, will connect to this server to evaluate their solutions and/or to apply their operators (single evaluation). If localeval is set to 1, one server instance per machine will be launched and the solutions will be evaluated locally (machine-level evaluation). Finally, if it is set to 2, the evaluation of solutions will also be performed locally but only one process per machine will be allowed (process-level evaluation). When the library is implemented in Java and this last mode of evaluation is used, the server as such disappears. So, if your library is implemented in this language, you must take this into account.
- typeexec: It is an argument that refers to the mode of execution of the library. It is checked when localeval is set to 1 or 2 (machine-level evaluation or process-level evaluation). It allows the seq, lan and wan values. If it is set to seq, the evaluation server will be launched in the local machine. Otherwise, a different instance of the server will be launched in every machine participating in the problem solution. If localeval is set to 0 (single evaluation), the value of this parameter is not taken into account because only one server instance will be launched.
- serverlocation: It is a string containing the name or the IP address of the machine in which the server will be launched. It is only checked when using single-evaluation mode (localeval=0).
- serverip: It is a string containing the IP address in which the server will await incoming connections. It is important not to mistake this parameter for the previous one. Although in most cases both parameters can take the same value, this is not always true. The toolbox may identify a machine with an IP address (an IP of a LAN, for example) and the server launched in that machine may accept connections in a different IP address (the real IP address of the machine outside the LAN, for example).
- serverevalport: It is a string containing an integer. It is the port in which the server will await requests concerning the evaluation of individuals and/or the application of operators.
- servercontrolport: It is a string containing an integer. It is the port in which the server will await requests concerning control commands. In the current implementation of the server this port is only used to receive the stop command.
- options: It is a cell matrix with options that this function may need to work. Table 3 shows both these options and when they are needed.
- origdir: It contains the path to a directory to go to in case of error.
Name | Needed if . . . |
mhtb.machines | localeval = 1 ∧ typeexec <> seq |
mhtb.machine.<i>.name | mhtb.machines > 0 |
mhtb_stopServers(localeval, typeexec, serverlocation, serverip, servercontrolport, options, origdir)
The arguments of this function have the same meaning as the ones received by mhtb_launchServers. If localeval is set to 1 or 2 (for C/C++ libraries) all the running
server instances need to be stopped. If localeval=0 only one server instance (located in serverlocation) needs to be stopped. To stop the servers, this function calls a Java class
contained in the same package as the server. This class connects with the server using serverip and servercontrolport, and sends it the stop string.
2. Functions to Handle the Configuration Parameters
Table 4 shows all the MATLAB functions belonging to this group. They will be explained with detail in this section.
Name | Description |
mhtb_readOptions | It reads the configuration parameters of the library from a file and stores them in a cell matrix. |
mhtb_writeOptions | It reads the configuration parameters of the library from a file and stores them in a cell matrix. |
mhtb_findOption | It searches a particular parameter in a parameters structure. |
mhtb_setOption | It sets the value of a particular parameter in a parameters structure. |
mhtb_removeOption | It removes a parameter from a parameters structure. |
mhtb_getKey | It returns the name of a parameter given its index inside a parameters structure. |
mhtb_getValue | It returns the value of a parameter given its index inside a parameters structure. |
mhtb_putPrefixToOptions | It appends the same prefix to all the parameters of a structure. |
mhtb_getOptionsWithPrefix | It returns the parameters of a structure with a given prefix. |
mhtb_isLabel | It returns ‘true’ if the value of a parameter is a tag. |
mhtb_substituteLabels | It replaces the values of tag parameters with their real values. |
[options] = mhtb_readOptions(file)
This function receives the path to a file containing configuration parameters with a suitable format and returns the same parameters stored in a cell matrix with as many rows as options there are in the file and two columns, the first one with the names of the parameters and the second one with their values.
- file: It contains the path to the file storing the parameters.
mhtb_writeOptions(structure, file, separator)
This function receives a parameters structure, the name of a file and a separating string. It writes this parameters in the file, one per line, with the option-separator-value format.
- structure : It is the structure the content of which we want to write into a file.
- file : It contains the path to the file to write the parameters into.
- separator : It is the separating string to be placed in the file between the name of each parameter and its value.
[found, value, index] = mhtb_findOption(structure, option)
This function obtains the value of a parameter inside a structure given its name. If the parameter is located, found will be set to ‘true’, value will contain the value of that parameter and index will return the index of that parameter in the structure. If the option is not located, found will be set to ‘false’.
- structure: It contains the structure in which we want to search for the parameter.
- option : It is the name of the parameter we want to search for.
[outstruct] = mhtb_setOption(structure, option, value)
This function allows us to establish the value of a parameter inside a parameters structure. The first step is to look for it within the structure. If it is found, its value is set to the desired value. If the parameter is not found, it is added with the given value. The function returns a rightly modified copy of the structure.
- structure: The structure in which we want to set a parameter.
- option: The name of the parameter the value of which we want to set.
- value: The value to which the parameter will be set.
[outstruct] = mhtb_removeOption(structure, option)
This function searches for a parameter in a structure given its name. If the parameter is found, this function returns a modified copy of the structure without the parameter. If not, it returns an error message.
- structure: It is the structure from which we want to remove a parameter.
- option: It is the name of the parameter we want to remove.
[k] = mhtb_getKey(structure, index)
This function allows us to obtain the name of a parameter given its index in a parameters structure. If this index if greater than the number of parameters contained in the structure, the function returns an error message.
- structure: It is the structure where we want to search.
- index: The index of the parameter in the structure the name of which we want to know.
[v] = mhtb_getValue(structure, index)
This function allows us to obtain the value of a parameter given its index inside the structure. If this index if greater than the number of parameters contained in the structure, the function returns an error message.
- structure: It is the structure where we want to search.
- index: The index of the parameter in the structure the value of which we want to know.
[outstruct] = mhtb_putPrefixToOptions(prefix, structure)
This function receives a parameters structure and adds the same prefix, followed by a dot, to all the parameters contained in it. The function returns a rightly modified copy of the structure.
- prefix: It is the prefix that will be added to all the parameters in the structure.
- structure: It is the structure we want to modify.
[outstruct] = mhtb_getOptionsWithPrefix(structure, prefix, rem_pref)
This function searches inside a parameters structure for all the parameters that begin with a given prefix and returns them stored inside a new parameters structure.
- structure: It is the structure in which they are to be searched for.
- prefix: The prefix to be searched for.
- rem_pref: if this parameter is set to ‘true’, the parameters in the new structure will not contain the prefix. Otherwise, the parameters will preserve their prefix.
[res] = mhtb_isLabel(label)
This function returns ‘true’ if the attribute is a tag. Otherwise, it returns ‘false’. The tags are delimited by < and >. For more information about the tags and their use in the configuration file, see the user’s guide.
- label: The attribute we want to check if it is a tag.
[outstruct] = mhtb_substituteLabels(structure, labels)
If you want to perform a parallel execution of any of the libraries in the toolbox, you need to configure all the machines taking part in the problem solution. Making a configuration file manually for each one of the machines is a tedious work and there is a lot of redundant information, so we offer a tags-based system. This system is explained in the user’s guide. What you need to know, as a developer, is that you can use this system when adding your own library. We encourage you to do so, because it makes the task of configuring the library much easier for the user.
This function receives a structure in which the values of some parameters contain tags to be replaced by their respective values and a second structure in which each one of the parameters contains a tag in its name.
It returns a new structure in which the tag-values in the first structure have been replaced by the values of the parameters in the second structure whose names contain the same tags. This process is clearly shown in the example in Table 5 .
Structure | Labels | ||
result.filename | <result> | <result> | onemax-lan.sol |
operator.4 | <recv> | <recv>.port | 3001 |
<recv>.max-con | 1 | ||
operator.7 | <send> | <send>.destinations | 1 |
<send>.destination.0.host | 192.168.3.2 | ||
<send>.destination.0.port | 3002 | ||
<send>.destination.0.try-time | 10000 | ||
Options | |||
result.filename | onemax-lan.sol | ||
operator.4.port | 3001 | ||
operator.4.max-con | 1 | ||
operator.7.destinations | 1 | ||
operator.7.destination.0.host | 192.168.3.2 | ||
operator.7.destination.0.port | 3002 | ||
operator.7.destination.0.try-time | 10000 |
- structure: structure with the tags we want to replace with values.
- labels: structure with the values to replace the tags with.
3. Auxiliary Functions
As regards the auxiliary functions, we are only going to mention one of them because of its usefulness:
[outstr] = substring(str, offset, len, repl)
This function shows a different behaviour depending on the number of arguments it receives. If it only receives three, it returns the substring of the first argument with the length indicated by the second one. It begins with an offset from the begining determined by the third argument.
If it also receives a fourth parameter, it does not return the substring determined by the three first one, but replaces it with this fourth parameter and returns the result.
- str: The string from which a substring will be extracted or replaced.
- offset: The offset from the begining of str.
- len: The length of the substring to be extracted/replaced.
- repl: The string to replace the str substring with.