In this section we are showing the interfaces that you need to follow when implementing your own objective functions and operators to solve a problem, and we illustrate them with examples.
1. Objective Functions
The objective functions receive a vector representing the evaluable part of a solution and return its fitness for the problem under consideration. Our toolbox solves maximization problems, that is, it considers that a certain solution is better than another if it has a higher fitness value. The interface for the objective functions is:
function y = objective(x) |
For example, if you want to solve the onemax problem, consisting in maximizing the number of ones in a binary string, you can implement the objective function shown below, which returns the sum of the vector that it has received as an argument:
function y = onemax(x) |
y = sum(x); |
The Frequency Modulation Sounds problem (FMS) consists in determining the 6 parameters a1,ω1,a2,ω2,a3,ω3 of the FM Sound model represented by
y(t) = a1 · sin(ω1 · t · θ + a2 · sin(ω2 · t · θ + a3 · sin(ω3 · t · θ)))with θ = (2 · π · 100). The fitness function is defined as the summation of square errors between the evolved data and the model data, as follows:
ffms(a1,ω1,a2,ω2,a3,ω3) = ∑ t=0:100 (y(t) - y0(t))2The model data are given by the following equation:
y0(t) = 1.0 · sin(5.0 · t · θ - 1.5 · sin(4.8 · t · θ + 2.0 · sin(4.9 · t · θ))).
Each parameter is in the range -6.4 to 6.35. This is a highly complex multimodal problem with a strong epistasis.
As our toolbox works solving maximization problems, the fitness function implemented in MATLAB is the reverse of the fitness function shown above. To avoid dividing by 0, we add 0.001 to the denominator, so the fitness value of the global minimum is 1000. The code of this function is shown in Figure 1.
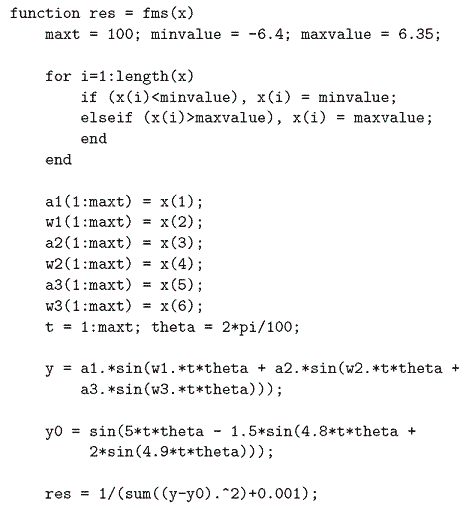
Figure 1: MATLAB function to solve the FMS problem
2.Operators
Because of the large amount of different operators that can be used, the interface for the MATLAB functions implementing them is very general. The data received by the functions depend strongly on the kind of operator we implement and the library we are using to solve the problem. The defined interface is the following:
function res = operador(inds, props) |
where inds is a MATLAB structure with the following fields:
- popl: The population size.
- data: A 2-dimensional matrix with popl rows. Each row stores the evaluable part of an individual in the population.
- sigma: A 2-dimensional matrix with popl rows. This field is only used in Evolutionary Strategies. Each row stores the standard deviations vector of one individual in the population.
- alpha: A 2-dimensional matrix with popl rows. This field is only used in Evolutionary Strategies. Each row stores the angles vector of one individual in the population.
- fit: A row vector with a length of popl containing the fitness values of all the individuals in the population.
- n_ind: The number of individuals received by the operator.
- data_op: A 2-dimensional matrix with n_ind rows. Each row stores the evaluable part of one individual received by the operator.
- sigma_op: A 2-dimensional matrix with n_ind rows. This field only makes sense in Evolutionary Strategies. Each row stores the standard deviations vector of one individual received by the operator.
- alpha_op: A 2-dimensional matrix with n_ind rows. This field is only used in Evolutionary Strategies. Each row stores the angles vector of one individual received by the operator.
- fit_op: A row vector with a length of n_ind containing the fitness values of all the individuals received by the operator.
When using the MALLBA library, the only fields that may have a content are n_ind, data_op,
sigma_op y alpha_op. In the jEA library, all the fields can be used. The ssGA library does not
admit the addition of new operators.
props contains the operator parameters defined by the user in the configuration file, stored
in a 2-dimensional cell array with as many rows as the number of parameters of the operator,
and two columns: the first one containing the name of the parameter and the second one its
value.
res must be a MATLAB structure with the following fields:
- n_ind: The number of individuals returned by the operator. Operators in the MALLBA library must return the same number of individuals they have received. There are no restrictions in the number of individuals returned by the operators in the jEA library.
- data: A 2-dimensional matrix with n_ind rows. Each file will store the evaluable part of one individual returned by the operator.
- sigma: A 2-dimensional matrix with n_ind rows. It is only used in Evolutionary Strategies. Each row will store the standard deviations vector of one individual returned by the operator.
- alpha: A 2-dimensional matrix with n_ind rows. It only makes sense in Evolutionary Strategies. Each row will contain the angles vector of one individual returned by the operator.
- fit: A row vector with a length of n_ind, containing the fitness values of all the individuals returned by the operator.
As an example, the code of a random selection operator is shown. This operator receives some individuals and returns as many as indicated by the select parameter, chosen at random. This function is shown in Figure 2.
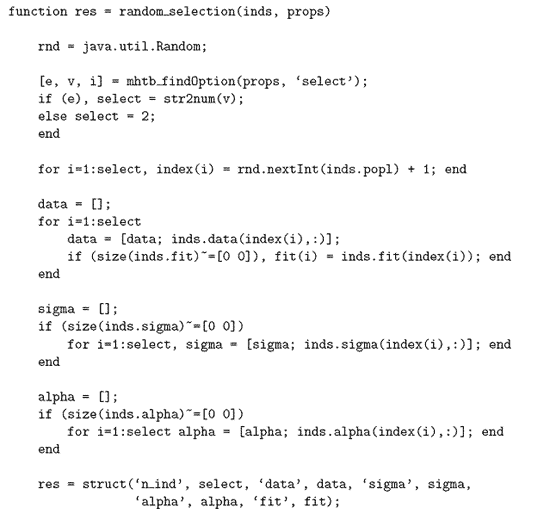
Figure 2: Random Selection operator